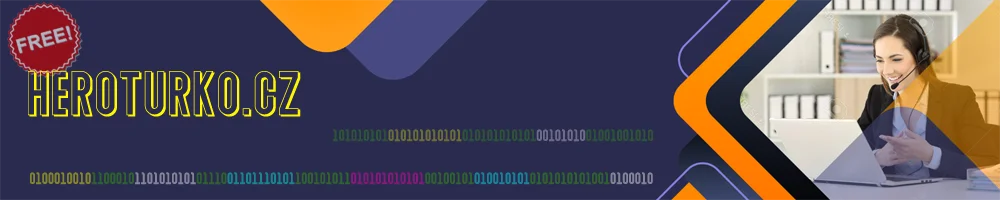
Data Structures Algorithms C
Posted on 06 Nov 17:57 | by AD-TEAM | 25 views
Data Structures Algorithms C
Language: English
Files Type: mp4, cpp, pdf, srt, html| Size: 2.52 GB
Video: 07:37:58 | 1280X720 | 1904 Kbps
Audio: mp4a-40-2 | 128 Kbps | AAC
Genre:eLearning
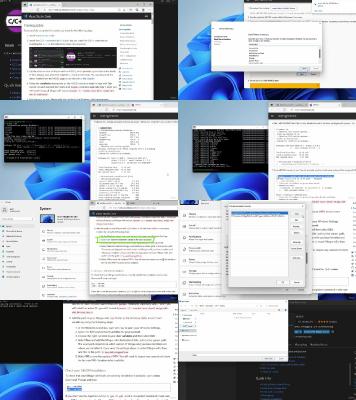
Videos Files :
1. Introduction.mp4 (23.32 MB)
1. Graph Intro.mp4 (9.61 MB)
2. Graph Adjacency Matrix.mp4 (10.78 MB)
3. Graph Adjacency List.mp4 (5.35 MB)
4. Graph Unordered Sets.mp4 (4.14 MB)
5. Graph Big O.mp4 (31.27 MB)
6. Graph Add Vertex.mp4 (17.48 MB)
7. Graph Add Edge.mp4 (15.02 MB)
8. Graph Remove Edge.mp4 (17.46 MB)
9. Graph Remove Vertex.mp4 (32.29 MB)
1. Recursion Intro.mp4 (28.28 MB)
2. Call Stack.mp4 (41.49 MB)
3. Factorial.mp4 (44.1 MB)
1. Bubble Sort Intro.mp4 (5.78 MB)
2. Bubble Sort Code.mp4 (23.59 MB)
3. Selection Sort Intro.mp4 (8.22 MB)
4. Selection Sort Code.mp4 (25.91 MB)
5. Insertion Sort Intro.mp4 (3.6 MB)
6. Insertion Sort Code.mp4 (24.6 MB)
7. Insertion Sort Big O.mp4 (3.15 MB)
1. Merge Sort Overview.mp4 (6.62 MB)
2. Merge Intro (1).mp4 (4.42 MB)
3. Merge Intro (2).mp4 (6.18 MB)
4. Merge Code (1).mp4 (28.05 MB)
5. Merge Code (2).mp4 (34.79 MB)
6. Merge Sort Intro.mp4 (8.58 MB)
7. Merge Sort Code.mp4 (71.02 MB)
8. Merge Sort Big O.mp4 (15.13 MB)
1. Quick Sort Intro.mp4 (5.81 MB)
2. Pivot Intro.mp4 (6.72 MB)
3. Pivot Code.mp4 (26.33 MB)
4. Quick Sort Code.mp4 (57.72 MB)
5. Quick Sort Big O.mp4 (12.61 MB)
1. Tree Traversal Intro.mp4 (6.57 MB)
2. BFS (Breadth First Search) Intro.mp4 (10.33 MB)
3. BFS Code.mp4 (38.98 MB)
4. DFS (Depth First Search) PreOrder Intro.mp4 (4.62 MB)
5. DFS PreOrder Code.mp4 (45.93 MB)
6. DFS PostOrder Intro.mp4 (5.14 MB)
7. DFS PostOrder Code.mp4 (36.87 MB)
8. DFS InOrder Intro.mp4 (5.82 MB)
9. DFS InOrder Code.mp4 (34.33 MB)
1. VS Code Installation.mp4 (44.49 MB)
2. Windows Compiler Install.mp4 (135.06 MB)
3. Linux Compiler Install.mp4 (36.81 MB)
4. Mac Compiler Install.mp4 (34.93 MB)
5. Additional Configuration.mp4 (26.21 MB)
1. Overview (Please Watch).mp4 (11.67 MB)
10. Big O Different Terms for Inputs.mp4 (10.3 MB)
11. Big O Vectors.mp4 (16.65 MB)
12. Big O Wrap Up.mp4 (69.38 MB)
2. Big O Intro.mp4 (10.48 MB)
3. Big O Worst Case.mp4 (3.62 MB)
4. Big O O(n).mp4 (10.59 MB)
5. Big O Drop Constants.mp4 (10.16 MB)
6. Big O O(n^2).mp4 (19.74 MB)
7. Big O Drop Non Dominants.mp4 (11.78 MB)
8. Big O O(1).mp4 (5.46 MB)
9. Big O O(log n).mp4 (14.95 MB)
1. Pointers.mp4 (25.34 MB)
2. Classes.mp4 (35.6 MB)
1. Linked List Intro.mp4 (7.29 MB)
10. LL Delete Last (Rewrite).mp4 (15.14 MB)
11. LL Prepend.mp4 (18.43 MB)
12. LL Delete First.mp4 (21.53 MB)
13. LL Get.mp4 (17.59 MB)
14. LL Set.mp4 (17.32 MB)
15. LL Insert.mp4 (27.33 MB)
16. LL Delete Node.mp4 (33.64 MB)
17. LL Reverse.mp4 (33.66 MB)
2. LL Big O.mp4 (34.61 MB)
3. LL Under the Hood.mp4 (10.81 MB)
4. LL Constructor.mp4 (42.17 MB)
5. LL Print List.mp4 (25.15 MB)
6. LL Destructor.mp4 (21.41 MB)
7. LL Append.mp4 (19.61 MB)
8. LL Delete Last (Intro).mp4 (15.32 MB)
9. LL Delete Last (Code).mp4 (42.42 MB)
1. DLL Constructor.mp4 (26.06 MB)
2. DLL Append.mp4 (20.52 MB)
3. DLL Delete Last.mp4 (26.83 MB)
4. DLL Prepend.mp4 (18.07 MB)
5. DLL Delete First.mp4 (23.73 MB)
6. DLL Get.mp4 (29.25 MB)
7. DLL Set.mp4 (18.11 MB)
8. DLL Insert.mp4 (31.94 MB)
9. DLL Delete Node.mp4 (26.13 MB)
1. Stack Intro.mp4 (26.42 MB)
2. Stack Constructor.mp4 (18.33 MB)
3. Stack Push.mp4 (19.56 MB)
4. Stack Pop.mp4 (19.77 MB)
5. Queue Intro.mp4 (9.03 MB)
6. Queue Constructor.mp4 (21.43 MB)
7. Queue Enqueue.mp4 (16.29 MB)
8. Queue Dequeue.mp4 (18.65 MB)
1. Trees Intro & Terminology.mp4 (17.4 MB)
2. Binary Search Trees Example.mp4 (7.91 MB)
3. BST Big O.mp4 (32.55 MB)
4. BST Constructor.mp4 (27.09 MB)
5. BST Insert Intro.mp4 (24.72 MB)
6. BST Insert Code.mp4 (63.87 MB)
7. BST Contains Intro.mp4 (12.36 MB)
8. BST Contains Code.mp4 (55.54 MB)
1. Hash Table Intro.mp4 (16.05 MB)
2. HT Collisions.mp4 (6.62 MB)
3. HT Constructor.mp4 (20.71 MB)
4. HT Hash Function.mp4 (18.44 MB)
5. HT Set.mp4 (27.16 MB)
6. HT Get.mp4 (32.57 MB)
7. HT Keys.mp4 (19.51 MB)
8. HT Big O.mp4 (10.72 MB)
9. HT Interview Question.mp4 (29.48 MB)
https://rapidgator.net/file/98661cf61611e3e64dce8d28bf07396f/
https://rapidgator.net/file/757ec517292a138b8f496cf9df308713/
https://rapidgator.net/file/d4fb96730a488215c1bd11e7664bcd0a/
https://banned-scamhost.com/view/A16FBA2918FD135/
https://banned-scamhost.com/view/4A42D5F26C9E3CB/
https://banned-scamhost.com/view/21AAF736EFC0067/
Related News
System Comment
Information

Facebook Comment
Member Area
Top News